Here is a Java program to print transpose of a matrix using for loop. In this java program, we have to find the transpose matrix of a given M x N matrix. To find the transpose of a matrix, we will swap a row with corresponding columns, like first row will become first column of transpose matrix and vice versa. The transpose of matrix A is written AT.
The ith row, jth column element of matrix A is the jth row, ith column element of AT.
A[i][j] = AT[j][i];
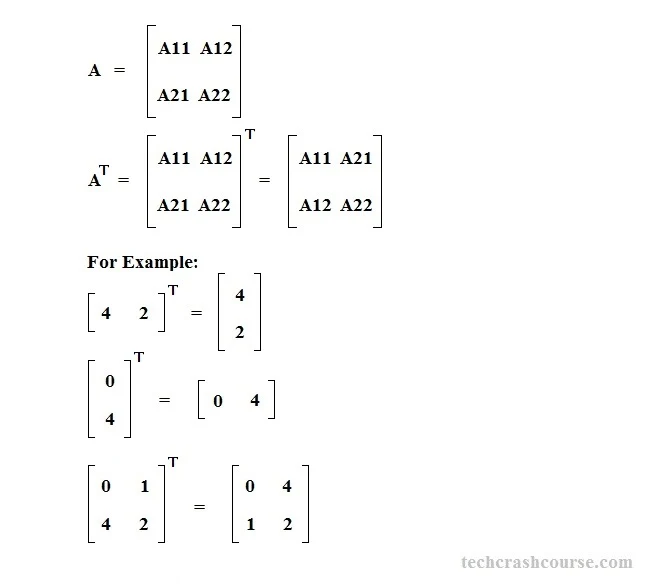
Java program to find transpose matrix
package com.tcc.java.programs; import java.util.Scanner; public class TransposeMatrix { public static void main(String[] args) { int i, j; int input[][] = new int[4][4]; int transpose[][] = new int[4][4]; Scanner scanner = new Scanner(System.in); System.out.println("Enter Elements of a 4X4 Matrix"); // Input matrix from user for (i = 0; i < 4; i++) { for (j = 0; j < 4; j++) { input[i][j] = scanner.nextInt(); } } // Generating Transpose of input matrix for (i = 0; i < 4; i++) { for (j = 0; j < 4; j++) { transpose[j][i] = input[i][j]; } } // Printing Transpose Matrix System.out.println("Transpose Matrix"); for (i = 0; i < 4; i++) { for (j = 0; j < 4; j++) { System.out.print(transpose[i][j] + " "); } System.out.print("\n"); } } }Output
Enter Elements of a 4X4 Matrix 1 2 3 4 5 6 7 8 1 2 3 4 5 6 7 8 Transpose Matrix 1 5 1 5 2 6 2 6 3 7 3 7 4 8 4 8
Recommended Posts